Lab 1.1: Introduction to python
Part of Week 1: Introducing pythonGeneral setup
For all lab exercises you should create a folder for the lab somewhere sensible.
Assuming you have a GAMR1520-labs folder, you should create a GAMR1520-labs/week_1 folder for this week and a GAMR1520-labs/week_1/lab_1.1 folder inside that.
Create a collection of scripts. If necessary, use folders to group related examples.
GAMR1520-labs └─ week_1 └─ lab_1.1 ├─ experiment1.py └─ experiment2.py
Try to name your files better than this, the filename should reflect their content. For example, string_methods_.py, conditionals.py or while_loops.py.
Make sure your filenames give clues about what is inside each file. A future version of you will be looking back at these, trying to remember where a particular example was.
General approach
As you encounter new concepts, try to create examples for yourself that prove you understand what is going on. Try to break stuff, its a good way to learn. But always save a working version.
Modifying the example code is a good start, but try to write your own programmes from scratch, based on the example code. They might start very simple, but over the weeks you can develop them into more complex programmes.
Think of a programme you would like to write (don't be too ambitious). Break the problem down into small pieces and spend some time each session trying to solve a small problem.
In this set of exercises we are assuming that you have no experience with python. We will begin by getting familiar with some of the basics using the interactive python interpreter. We will cover some fundamental aspects of python in isolation. We will then move on to putting them together into short python scripts.
Following these exercises will help to develop some experience with the workflow involved in writing python code and to become familiar with how terms such as literals, variables, types and operators apply in a practical setting.
Table of contents
- Getting started with IDLE
- Basic principles
- Writing larger programmes
- Conditionals
- Obtaining user input
Getting started with IDLE
To get started, we will work with Python’s Integrated Development and Learning Environment (IDLE).
IDLE is not a great code editor but will be useful for this introduction to the language.
Open IDLE on your machine. It should look something like this.
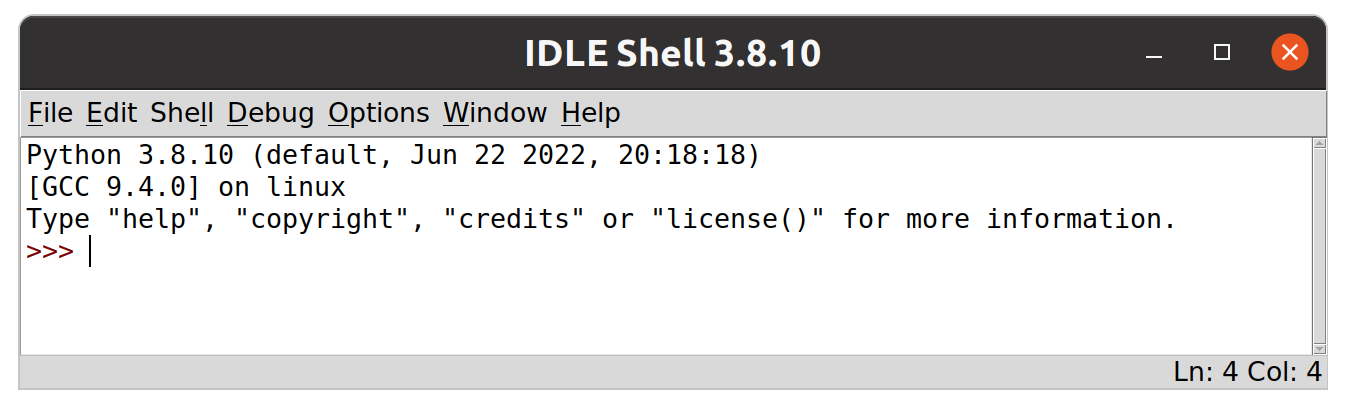
It may not look exactly like this. These screenshots were created on a linux machine. It shouldn’t matter what OS you are running. On Windows and MacOS IDLE is usually installed along with python. On Linux distributions you may need to install it separately.
Your python version may also be different. My system python was 3.8 when I took this screenshot. I have now upgraded to 3.10 which matches the 2022 lab version. Hopefully you have a more recent version.
The main IDLE window provides an interactive python shell. The prompt allows the user to enter any valid python statement. Each expression entered will be evaluated and the result printed before another prompt will appear, waiting for the next statement. This kind of interface is known as a Read, Execute, Print Loop or REPL.
Basic principles
Let’s get to grips with some very basic python expressions.
The following terms are used in most programming languages, but the details of each language are different.
Literals
We will begin with literal values.
These are hard-coded values expressed directly in your source code, such as 1
, True
or 'hello'
.
When literal values are evaluated by python, they evaluate to themselves.
123
Entering the above into IDLE will produce output like this:
123
Practice using literals
Read about the concept of literals in python. Practice entering literal expressions into IDLE. Make sure you understand what is going on.
Operators
Literal values are great, but they are not much use on their own. We often want to transform data using operators.
Common operators include +
, -
, /
etc.
We can use operators to combine literals, and generate new values.
123 + 1
Entering the above into IDLE will produce output like this:
124
Read through the operators page and try all the examples.
Practice using operators
Read about operators in python. Try different combinations of operators. See what errors you can cause. Check to see if you understand what is going on.
- Perform some calculations
- Perform some string manipulation
- Cause a TypeError by combining different data types
Variables
Manipulating data is limited without variables to store data.
We can use the assignment operator (=
) to assign values to variables.
a = 123
Entering the above into IDLE will produce no output. To create output, we can enter another simple expression.
a + 1
The result is as expected.
124
Read through the variables and assignment page and try all the examples.
Keep experimenting
In the next section we are going to start working with larger programmes. However, you can write simple programmes in the IDLE interactive prompt.
- Experiment with assigning values to variables.
- Use expressions which combine literals and operators.
- Enter expressions which combine your variables further.
- e.g. calculate the surface area and circumference of a circle with a given radius.
- Raise a NameError.
Writing larger programmes
So far we have been looking at very simple, one-liner programmes. At some point we need to move beyond this and write programmes consisting of several steps.
Managing source code
In order to write longer programmes with multiple statements, we need to move from the interactive interpreter to working with source code files.
Programmes written in this way won’t automatically output the evaluated result of each line like the shell does. One way to generate output in the shell is to explicitly use the built-in
print()
function.
Using the file menu in IDLE, go to file -> new file (or use the shortcut Ctrl + N) to open a new IDLE file window.
There should now be two IDLE windows open; the interpreter (or shell) and a new, empty file window, ready to receive code.
Type this into the file window.
print('Hello world')
Be careful to enter this into the file window, not the shell window
Anything passed into the print()
function will be automatically converted to a string and outputted to the terminal.
Now save your programme to a sensible location on your filesystem using the file -> save option (or Ctrl + S). It is recommended that you create a folder for today’s work (probably inside a folder for the module) and save your code in there.
A folder structure like this for example
GAMR1520-labs └── week_1 ├── lab_1.1 │ ├── experiment1.py │ └── experiment2.py │ ├── lab_1.2 │ ├── experiment3.py │ └── experiment4.py │ └── lab_1.3 ├── experiment5.py └── experiment6.py
Obviously, your own experiments can be named to indicate what they contain. Feel free to use any sensible system you like, just make sure you keep examples of working code and add comments to the files as necessary.
Try to avoid spaces or hyphens in your file names, underscores are fine. For example, this file could be named hello_world.py
In IDLE, files can be executed via the Run menu. Go to Run -> Run Module (or use the shortcut key F5) to execute our first python programme.
A python file is known as a module. A folder containing a collection of modules is known as a package.
You should see the code runs in the IDLE shell and outputs our message.
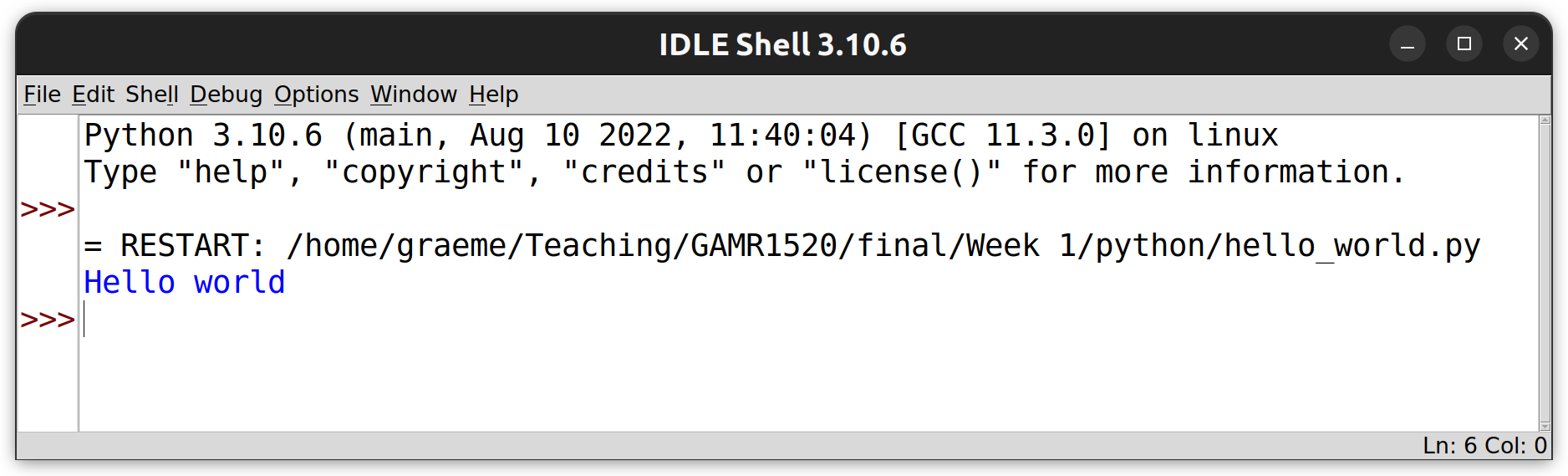
Did you notice, I upgraded my python version!
Write a script
Try writing some simple programmes of your own that combines everything you have learned so far.
Create new files and keep your experiments for later reference.
Conditionals
Now we can write more complex programmes, we need a way to control the flow of execution. In Python, the main way we can do this is via Compound statements.
The simplest example of a compound statement is probably a conditional.
Conditional statements allow us to make decisions in our code based on Boolean values.
This is achieved using if
, else
and elif
keywords.
The following code demonstrates a simple if
clause.
if balanceA >= amount:
balanceA -= amount
balanceB += amount
You should have seen this code before. If not, have a look at compound statements before continuing.
Notice that the conditional statement includes a comparison operator. This is one very common ways to use a conditional statement. But any expression that resolves to a Boolean value is fine.
Notice also that the if
line ends with a colon and that after the if
line, there is an indented code block.
The code block could contain any number of lines, but in this case it contains only two lines.
The code is moving funds from one account to another.
The header checks whether balanceA
is large enough to make the move.
The code block reduces balanceA
and increases balanceB
.
The behaviour of this code will depend on the value of the variables.
If balanceA
is less than the required amount
, then the block will be skipped and both balances will be unaffected.
However, if balanceA
is greater than or equal to the required amount
then the block will be executed and the balances will be affected accordingly.
Note that, if any of these variables was undefined, the code would raise a NameError
and, if the variables were of a type that cannot be compared with or added to each other (e.g. a string and an integer), the code would raise a TypeError
. These errors would crash the programme.
Complete the code
Write the following code into a file balance.py
if balanceA >= amount: balanceA -= amount balanceB += amount
Expand the programme by declaring the variables and giving them initial values. Add some
print()
statements to generate output. Try a few values for the variables to see what you get. Can you produce errors by assigning the variables to data of the wrong types?Remember, in IDLE you need to save code with Ctrl + S and press F5 to execute.
Adding an else
clause
Conditionals can be extended with an else
clause to provide another code block to execute only if the condition fails.
With this kind of conditional, we can be sure that one or other of the code blocks will execute, but never both.
Here is an extended programme to which we have added a simple message to indicate that the conditional test failed.
balanceA = 100
balanceB = 50
amount = 10
if balanceA >= amount:
balanceA -= amount
balanceB += amount
else:
print("Insufficient funds!")
print("Balance A: ", balanceA)
print("Balance B: ", balanceB)
What’s going on here?
Can you see which lines of code execute and when?
Remember, indentation is important.
What happens if we set amount
to 60
or balanceA
to 0
?
Let’s review what we have learned with another example.
Create a new file with Ctrl + N and add the following code.
a = 10
b = 20
if a > b:
print('a is larger than b')
else:
print('b is larger than a')
Save the above code as conditionals.py and press F5 to execute.
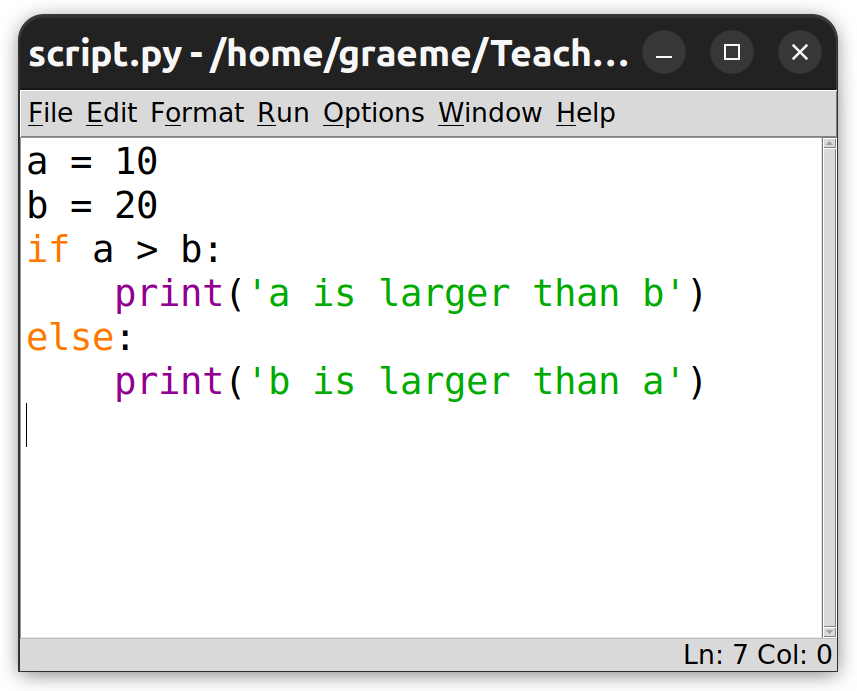
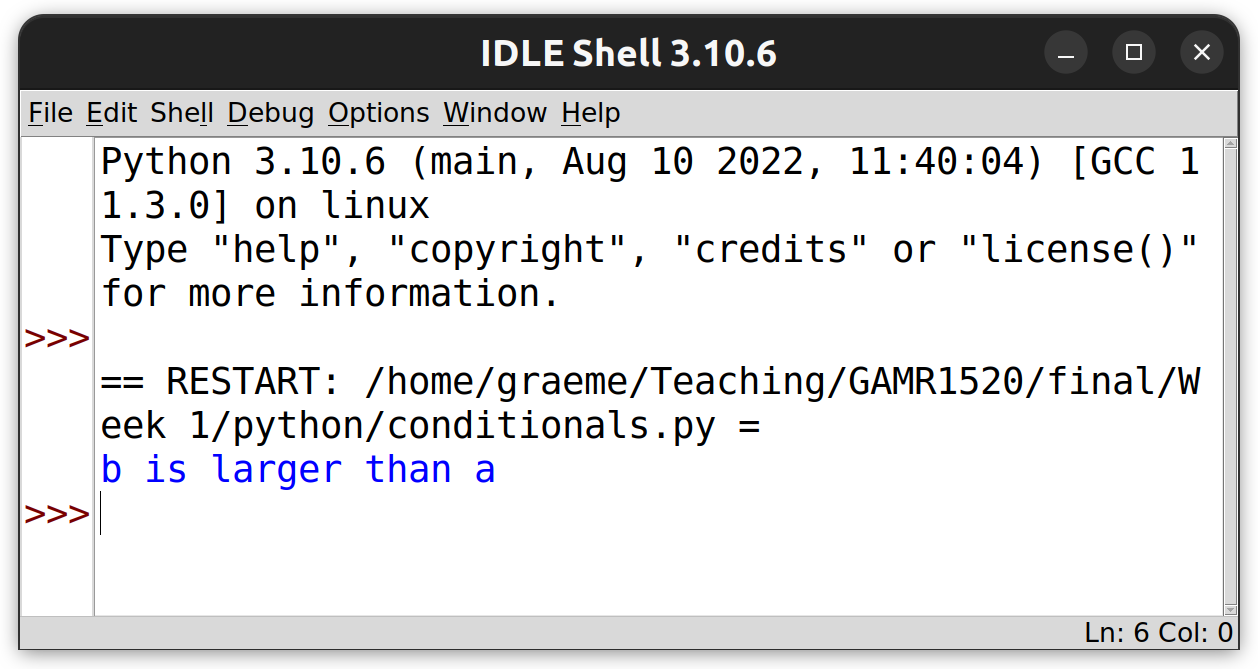
Be careful not to confuse the interpreter window with the script window. The interpreter window has
>>>
prompts. The script window should show only your code.
Can you see a flaw in the above code?
What happens when
a
andb
are equal?It’s also possible to add
elif
clauses betweenif
andelse
clauses. Write an improved programme which handles all three cases using anelif
clause. Use the python documentation for help.
Obtaining user input
We have already seen how we can use the built-in print()
function to generate output from our scripts.
If we want to take user input, we can use the input()
function.
The built in input()
function is a simple way to get input from users via the terminal/shell/command prompt.
When we call input()
, our programme will wait to allow the user to type something and press enter.
Once the user enters a value, the input()
function returns a string containing whatever the user typed.
You need to go to the IDLE shell window to enter some input. If the user does not press enter, the programme will wait forever.
The input()
function can take an optional argument which it will output as a prompt for the user.
Create a new file greeting.py and try the following.
name = input('Enter your name: ')
print('Hello ' + name)
This allows us to write scripts that interact with the user!
Now, we can experiment with our balance.py script to make it more interactive. We want to display the balances so the user can decide how much they want to transfer, and then display the resulting balances.
If you closed the file, use the file -> open menu option to reopen it.
Here’s some new code:
There is a bug which will present itself if you run the code. Look closely, can you find the bug before running it? It’s not obvious.
balanceA = 10
balanceB = 0
print("Balance A: ", balanceA)
print("Balance B: ", balanceB)
amount = input("Amount to transfer from A to B:")
if balanceA >= amount:
balanceA -= amount
balanceB += amount
else:
print("Insufficient funds!")
print("Balance A: ", balanceA)
print("Balance B: ", balanceB)
Consider what the variable amount
will equal and how it is used in the conditional statement.
We are using a comparison operator to compare balanceA
with amount
.
Since the amount
variable is assigned to the result of a call to input()
, it will contain a string.
The user may enter the string '5'
or 'banana'
, either way, it’s still a string.
To do the comparison, we need to cast the result to a number (either using float
or int
, depending on what we want).
Fix the programme by adding this line.
amount = float(amount)
It needs to be added after the input()
line and before the comparison.
Alternatively, the entire call to
input()
can be wrapped in a call tofloat()
.amount = float(input("Amount to transfer from A to B:"))
Develop your existing examples
Upgrade your favourite scripts with some user input.
Wherever you have string literals, consider replacing them with user input. It won’t always be appropriate.
If you have integer or float literals, can you convert input strings to the right data type?